Read battery
Overview
Read the battery voltage using the AD converter on the CR2032 coin cell leaf.
This sample will also work with battery leaves such as the AA BAT leaf and the 2V to 4.5V leaf.
Leaf to use
Use the following leaves.
Type | Name | Q’ty |
---|---|---|
AZ62 | Connector Cover | 1 |
AZ01 | USB | 1 |
AP01 | AVR MCU | 1 |
AV01 | CR2032 | 1 |
CR2032 coin cell battery | 1 | |
M2*10mm screw | 2 |
Assembly
Source code
Write the following program in the Arduino IDE.
//=====================================================================
// Battery Voltage
//
// (c) 2020 Trillion-Node Study Group
// Released under the MIT license
// https://opensource.org/licenses/MIT
//
// Rev.00 2020/05/05 First release
//=====================================================================
#include <Wire.h>
const int BATT_ADC_ADDR = 0x50;
void setup() {
// initialize serial communication at 115200 second per second:
Serial.begin(115200);
// initialize I2C communication at 100kHz:
Wire.begin();
delay(10);
}
void loop(){
// read ADC registers:
Wire.beginTransmission(BATT_ADC_ADDR);
Wire.write(0x00);
Wire.endTransmission(false);
Wire.requestFrom(BATT_ADC_ADDR,2);
uint8_t adcVal1 = Wire.read();
uint8_t adcVal2 = Wire.read();
// when ADC is not connected, read values are 0xFF:
if (adcVal1 == 0xff && adcVal2 == 0xff) {
adcVal1 = adcVal2 = 0;
}
// voltage mV = adcVal * Vref(3.3V) / resolution(8bit) * Vdiv(2)
double tempMillivolt = ((double)((adcVal1 << 4) | (adcVal2 >> 4)) * 3300 * 2) / 256;
float dataBatt = (float)(tempMillivolt / 1000);
Serial.println("Batt[V] = " + String(dataBatt));
delay(1000);
}
Execution Results
Open the serial monitor of the Arduino IDE and set the baud rate to 115200 bps and you will see the battery voltage.
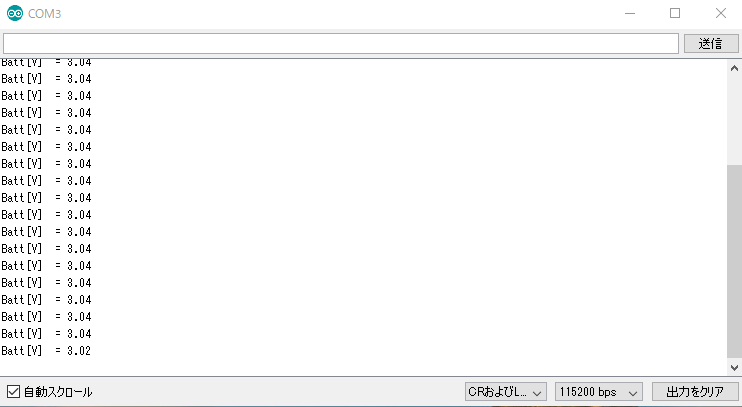
Last modified 15.03.2021