Temperature and humidity sensor
Overview
This sample reads the value of the temperature and humidity sensor mounted on the 4-Sensors leaf and displays it on the serial monitor.
Leaf to use
Use the following leaf.
Type | Name | Q’ty |
---|---|---|
AZ62 | Connector Cover | 2 |
AI01 | 4-Sensors | 1 |
AX07 | Back to back | 1 |
AP02 | ESP32 MCU | 1 |
AV04 | 2V~4.5V | 1 |
AZ63 | Nut Plate | 2 |
AAA battery holder | 1 | |
AAA battery | 3 | |
M2*8mm screw | 2 | |
M2*12mm screw | 2 | |
φ10x2mm magnet | 1 |
Assembly
Let’s assemble leaves as shown in the figure below.
Source code
Write the following program in the Arduino IDE.
In order to make this sketch work, you need to install the libraries If you haven’t installed it yet, refer to Environment to install it.
//=====================================================================
// Thermo-hygrometer ESP32
//
// (c) 2020 Trillion-Node Study Group
// Released under the MIT license
// https://opensource.org/licenses/MIT
//
// Rev.00 2020/07/28 First release
//=====================================================================
#include <Wire.h>
#include <HTS221.h>
//---------------------------
// Data for two-point correction
//---------------------------
// Temperature correction data 0
float TL0 = 25.0; // 4-Sensors Temperature measurement value
float TM0 = 25.0; // Thermometer and other measurements value
// Temperature correction data 1
float TL1 = 40.0; // 4-Sensors Temperature measurement value
float TM1 = 40.0; // Thermometer and other measurements value
// Humidity correction data 0
float HL0 = 60.0; // 4-Sensors Humidity measurement value
float HM0 = 60.0; // Hygrometer and other measurements value
// Humidity correction data 1
float HL1 = 80.0; // 4-Sensors Humidity measurement value
float HM1 = 80.0; // Hygrometer and other measurements value
void setup() {
// initialize serial communication at 115200 bit per second:
Serial.begin(115200);
// I2C 初期化
pinMode(21, OUTPUT); // SDA
digitalWrite(21, 0);
Wire.begin(); // I2C 100kHz
// initialize i2c communication with HTS221:
smeHumidity.begin();
delay(10);
}
void loop() {
// read temperature and humidity:
float dataTemp = (float)smeHumidity.readTemperature();
float dataHumid = (float)smeHumidity.readHumidity();
// calibration:
dataTemp = TM0 + (TM1 - TM0) * (dataTemp - TL0) / (TL1 - TL0); // 温度補正
dataHumid = HM0 + (HM1 - HM0) * (dataHumid - HL0) / (HL1 - HL0); // 湿度補正
Serial.println(String(dataTemp) + "[℃], " + String(dataHumid) + "[%]");
delay(1000);
}
2 point-to-point correction
If there is a discrepancy between the temperature (or humidity) that you want to display and the temperature (or humidity) of the 4-Sensors, perform the correction between the two points. The following is a description of the correction method using temperature as an example.
First, measure the temperatures of the two points with the 4-Sensors and a reference instrument. Then, write the measured values in the sample sketch and run it, and the temperature of the 4-Sensors will be close to the temperature you want to display.
Execution Results
Open the serial monitor in the Arduino IDE and set the baud rate to 115200 bps to display the temperature and humidity.
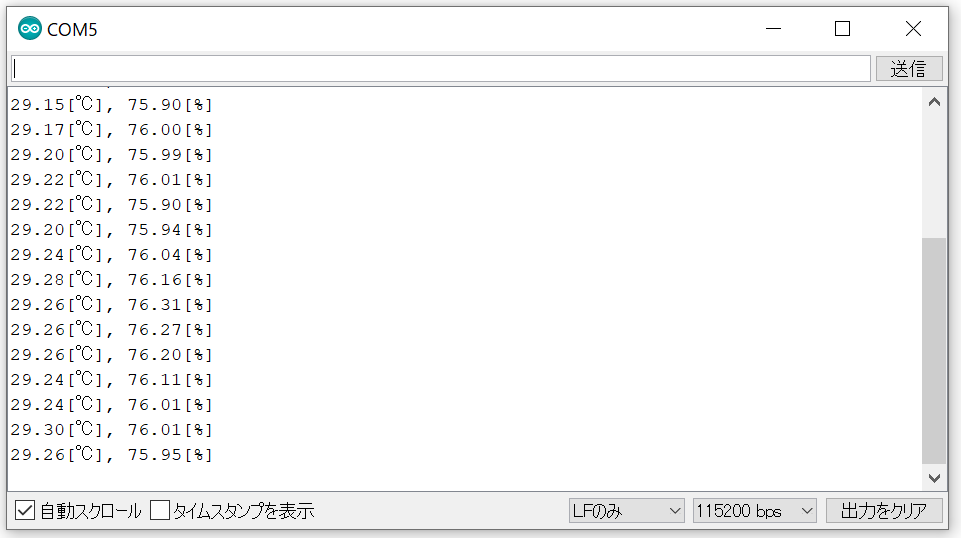